Biscuit Authorization Part II
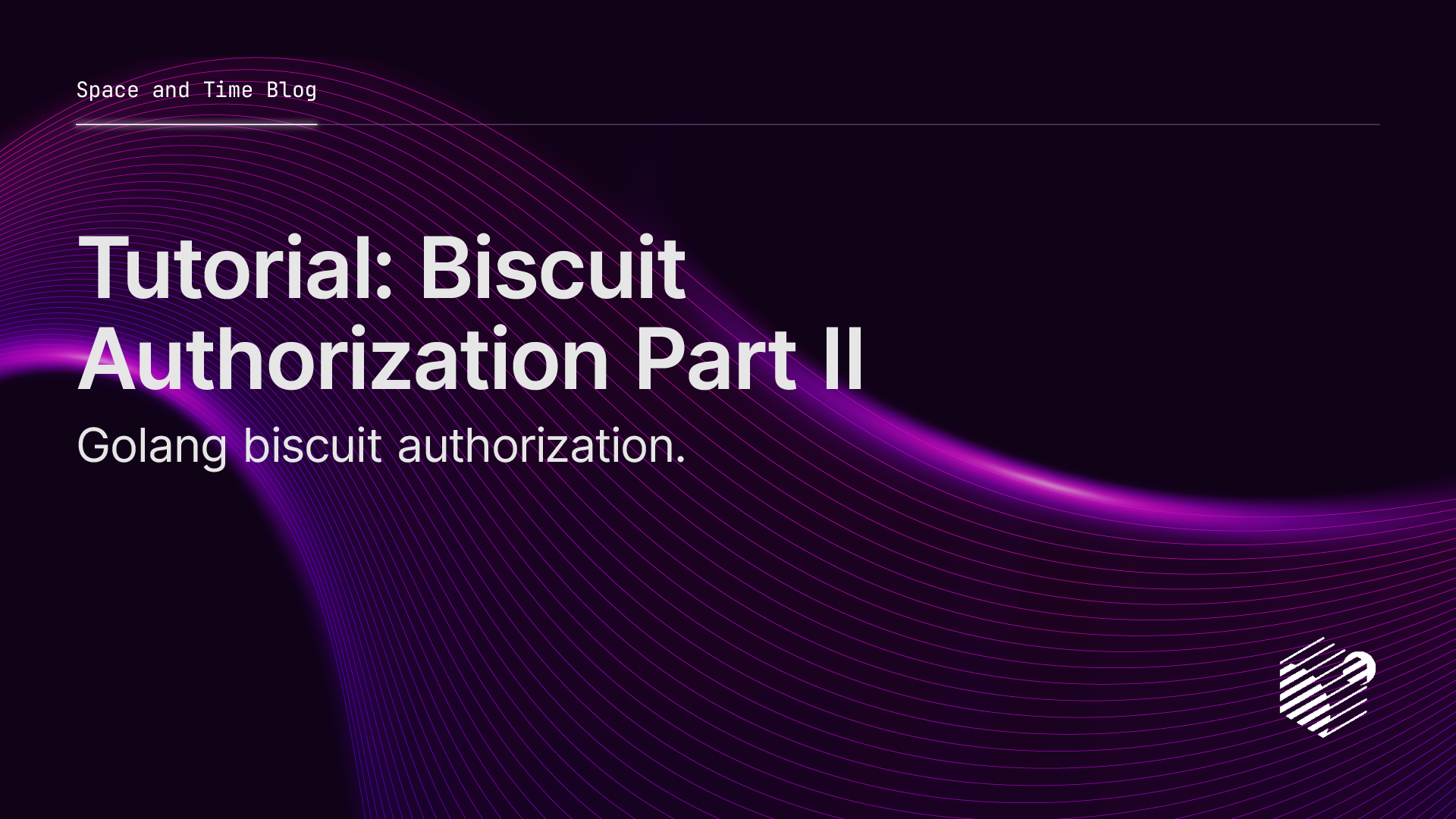
In Part 2 of the tutorial series, we will learn about Golang Biscuit authorization. Here, we will learn how to create, validate, attenuate, seal, and revoke the biscuit tokens. Let’s see how this works:
The tutorial series covers:
- Part 1: This tutorial covered the basics of Biscuits, creating a biscuit with a Command Line interface (CLI), and checking the authority of the token. We also covered the attenuation of the Biscuit tokens. Check out the tutorial here.
- Part 2: You are here. This tutorial covers how to use Biscuits with Golang.
Importing the Biscuit library
In this section, we will import the biscuit library into our Golang project. Let’s begin by running the following commands:
The commands above create golang-biscuit directory along with creating a go.mod file containing project dependencies. We also imported the biscuit-go library to our project. As of writing, the current version of the library is v2.1.0. The biscuit-go library has an internal dependency on Google Protocol Buffer library which is also imported. This is a cross-platform open-source library to serialize structured data. Now let’s dive into the actual code.
Creating Biscuit Token
Biscuit authorization needs the ED25519 library and public key cryptography to generate and verify the tokens. We will use the library to generate our private and public keys. The private key is required to generate the token, whereas the validation requires a public key. The following code shows how to generate our keys:
Now that we have our keys, let’s see how we can create our biscuit token:
In line 1, we created a new biscuit builder using the biscuit.NewBuilder(privateKey)method which implements a builder interface.
Next, we created a new fact from a string using parser.FromStringFact(`user("admin")`) where we instructed our program to create a new fact for the biscuit, i.e user(“admin”). Then we added the fact to our biscuit builder using builder.AddAuthorityFact(fact1). If you want to add multiple facts to the biscuit token, you can do it using the following:
After the facts are added, we actually created our biscuit usingbuilder.Build(). This generated our first biscuit. We converted it to a slice of bytes to make it shareable across our program using b.Serialize().
Important: Biscuit token compatibility across programming languages
It is important to remember that if a biscuit token is to be shared to other platforms using different programming languages, share a token that is base64 encoded. Otherwise, it won’t be recognized.
Validate a Biscuit token
In this section of Golang biscuit authorization, we will learn how to validate a biscuit token. This step includes creating an authorizer which contains a list of facts from the system and checks to allow or deny the incoming biscuit. The facts and rules are validated against the authorizer before it is allowed to access the requested resource. Here is a sample validator code:
The first line in the code above unmarshals the []byte biscuit token to *biscuit.Biscuit using biscuit.Unmarshal(biscuitToken). Remember, our biscuit token was serialized when it was created.
Then we created our authorizer with the public key, obtained above, using b.Authorizer(publicKey). We then list all our facts from the system (a database or any other source) and create biscuit facts for authorizer. In our case, we hardcoded two biscuit facts for the sake of this code, after which the facts are passed to the authorizer like this:
The next step involves adding a policy to the authorizer to allow or deny an incoming biscuit when the facts are matched. This is done with authorizer.AddPolicy(biscuit.DefaultAllowPolicy) or authorizer.AddPolicy(biscuit.DefaultDenyPolicy). In our case, we allowed the incoming biscuits that match the authorizer facts.
Finally, authorizer.Authorize() loops across all the facts and checks in the authorizer and matches against the same in the biscuit. If the checks are matched, the biscuits are allowed to access the required resource.
Attenuating a Biscuit
Attenuation is the process of generating a new biscuit token from an existing token by adding new checks. The new attenuated biscuit token will always have fewer access rights than the original biscuit token. Here is a sample code for attenuation:
Again, the first step is to obtain a *biscuit.Biscuit token from the passed serialized value which is done using biscuit.Unmarshal(biscuitToken).
After that, we added a new rule (check) in our existing biscuit to attenuate it using parser: FromStringCheck(`check if operation("create")`). This tells the attenuated biscuit token to check if the authorizer contains a fact named operation(“create”).
We know from Part 1 of the tutorial that the attenuated tokens contain extra blocks which hold the information on new rules. This new block is apart from the default authority block inside every token. So this new block is added using blockBuilder := b.CreateBlock() and the new check is attached to the block using blockBuilder.AddCheck(check1).
Lastly, we added the new block to the existing biscuit block using b.Append(rand.Reader, blockBuilder.Build()) and generated a new attenuated biscuit token. The attenuated token was serialized to be passed on to others.
Sealing Biscuits
Sealing is used to stop further attenuation of biscuit tokens. This is fairly easy to do with the code below:
In the above code, after obtaining the biscuit, we sealed the token using b.Seal(rand.Reader). This process again generated a new sealed biscuit token.
Revoking Biscuit Authorization Tokens
Sometimes we need to revoke a token from the system untimely if a token is compromised or a user is kicked out of the system. Then revocation comes into play.
The biscuit library provides us with a mechanism to list the revocation IDs in a token. But writing code to validate biscuits and maintaining a list of revoked tokens is the responsibility of the project. The list of revocation IDs to be maintained can be stored in a database, environment variable, or any other object storage medium and fetched dynamically in the authorizer.
We will use the same code from “Creating Biscuit Token” to generate a new token. Then we will fetch the revocation ID from the token and save it in an environment variable. Later on, we will use the same revocation ID to invalidate a token using our custom code.
The revocation ID of a biscuit token can be obtained and saved to the environment variable as follows:
The line revokationIds := b.RevocationIds(); generates [][]byte. This is because an attenuated token can have multiple revocation IDs equivalent to the number of blocks. We will revoke a simple biscuit token and hence, we are interested in the first revocation ID of the token only. That can be obtained using revokationIds[0]
The next step will be to add a new code block to our validator described in “Validate a Biscuit Token” just after obtaining our biscuit token. Add the following:
The above code checks if revocationId set in the environment variable matches the incoming biscuit’s revocation ID. If that matches, then the code throws an error.
Conclusion
In this tutorial, you have learned about Golang biscuit authorization. I have tried to cover all the basics of validations, checks, and facts. To learn more about these topics, you can visit this link.